PYQT5 Change QPushButton Text and Icon color on hover
By
John Phung
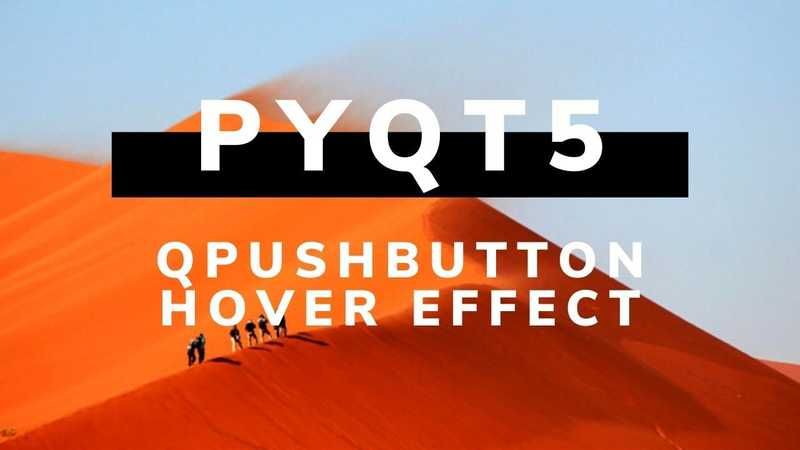
Change QPushButton Text and Icon Color on Hover
There was more struggle than expected to figure out how to achieve a simple hover color effect for a QPushButton using QT5, everything from using border-image or qproperty-icon properties in qss stylesheet. It turns out, you need to overwrite the QPushButton class by using event filtersĀ Mind Blown.
By subscribing to event filters in a newly construct QPushButton class, we can listen to the mouse events and the one we're interested is in the HoverEnter and HoverLeave events which I believe are enum values.
Below is the simple code that inherits the QPushButton class and changes the icon colour.
1class HoverButton(QPushButton):2 def __init__(self, title, icon_path, icon_path_hover, parent=None):3 super(NavButton, self).__init__()4 self.setText(title)5 self.setObjectName(title)6 self.defaultIcon = QIcon(resource_path(icon_path))7 self.hoverIcon = QIcon(resource_path(icon_path_hover))8 self.setIcon(self.defaultIcon);9 self.setIconSize(QSize(40, 20));10 self.setCursor(QCursor(Qt.PointingHandCursor))11 self.installEventFilter(self)1213 def eventFilter(self, source, event):14 if event.type() == QEvent.HoverEnter:15 self.setIcon(self.hoverIcon)16 self.setIconSize(QSize(40, 20));17 elif event.type() == QEvent.HoverLeave:18 self.setIcon(self.defaultIcon)1920 return super().eventFilter(source, event)
For changing the text colour we can simply use css by referencing the objectName and hover state and set the colour to something different.
In style.qss
1QPushButton#title {2 color: white;3}45QPushButton#title:hover {6 color: black;7}
Who knew such a simple UI effect requires so much work...